More calculations
By now we have been only calculating properties “on point”, but we can do more than that with yaeos
. Here we have a few examples:
Calculating an isotherm
Let’s create a plot Pressure vs Volume at a given temperature (250 K) for a pure component. Let’s use carbon dioxide.
[1]:
from yaeos import SoaveRedlichKwong
import numpy as np
import matplotlib.pyplot as plt
n = [1.0] # mole number of CO2 [mol]
Tc = [304.2] # critical temperature of CO2 [K]
Pc = [73.8] # critical pressure of CO2 [bar]
w = [0.2236] # acentric factor of CO2 [-]
# Instantiate a Soave-Redlich-Kwong equation of state model
model = SoaveRedlichKwong(Tc, Pc, w)
# Calculate isotherm at 250 K
t = 250 # K
# pressures list (200 pressure points between 5 and 30 bar)
pressures = np.linspace(5, 30, 200)
# list to store the calculated volumes
volumes = []
for p in pressures:
# Calculate volume for each pressure
volume = model.volume(n, p, t, root="stable")
volumes.append(volume)
# Make plot
plt.plot(volumes, pressures)
plt.xlabel("Volume [L]")
plt.ylabel("Pressure [bar]")
[1]:
Text(0, 0.5, 'Pressure [bar]')
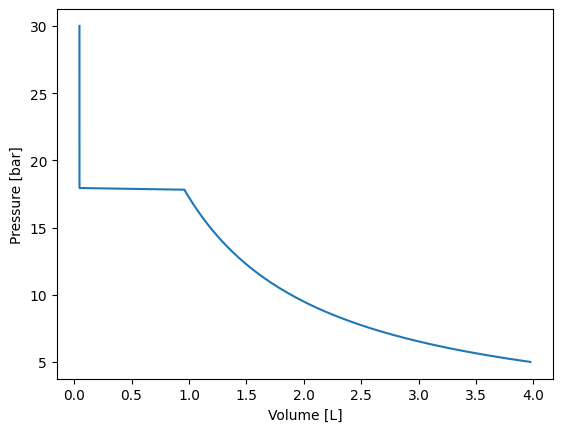
Calculate a saturation pressure
Pure compound
In the previous example we obtained an isotherm for carbon dioxide at 250 K. We can check in the plot that the saturation pressure is around 18 bar. Let’s calculate the previous saturation pressure using yaeos
and the instantiated model
.
[2]:
sat_point = model.saturation_pressure(z=[1.0], temperature=250.0)
sat_point
[2]:
{'x': array([1.]),
'y': array([1.]),
'Vx': 0.04668949889514571,
'Vy': 0.9534768478027397,
'T': 250.0,
'P': 17.916706382441067,
'beta': 0.0}
There we obtained a Python dictionary with the result of the saturation pressure calculation. In this example we have a pure compound (CO2), so we are only interested in the P
result (the saturation pressure).
[3]:
print(sat_point["P"], " bar")
17.916706382441067 bar
The DIPPR correlated value for the saturation pressure of CO2 at 250 K is 17.88 bar, so SoaveRedlichKwong is doing a good job in this case.
Mixture
Of course, in thermodynamics there is not only pure compounds, we can also use yaeos
to calculate the saturation pressure of a mixture. Let’s calculate the saturation pressure of a mixture of 50% CO2 and 50% n-butane at 250 K. But now let’s use PengRobinson78
.
[4]:
from yaeos import PengRobinson78
# Pure compounds properties
n = [0.5, 0.5] # mole number of CO2 and n-butane [mol]
Tc = [304.2, 425.1] # critical temperature of CO2 and n-butane [K]
Pc = [73.8, 38.0] # critical pressure of CO2 and n-butane [bar]
w = [0.2236, 0.200164] # acentric factor of CO2 and n-butane [-]
model = PengRobinson78(Tc, Pc, w)
# Calculate bubble point pressure
bubble_point = model.saturation_pressure(z=n, temperature=250.0, kind="bubble")
# Calculate dew point pressure
dew_point = model.saturation_pressure(z=n, temperature=250.0, kind="dew")
Let’s check our results:
[5]:
bubble_point
[5]:
{'x': array([0.5, 0.5]),
'y': array([0.96800305, 0.03199695]),
'Vx': 0.06509074642358809,
'Vy': 2.2963934569223667,
'T': 250.0,
'P': 8.26969042084664,
'beta': 0.0}
[6]:
dew_point
[6]:
{'x': array([0.02630095, 0.97369905]),
'y': array([0.5, 0.5]),
'Vx': 0.08695218909925097,
'Vy': 26.111736032213937,
'T': 250.0,
'P': 0.781612858279228,
'beta': 1.0}
In both calculations we have similar information. We have the composition of each phase x
and y
. In the case of bubble point the composition x
is the same as the global composition that we provide as input, of course. And the y
are the compositions of the vapor phase. In the case of the dew point the composition y
is the same as the global composition that we provide as input, and the x
are the compositions of the liquid phase.
yaeos
give us the molar volume of each phase Vx
and Vy
in [L / mol].
Then we got the temperature (same value that we provide for the calculation). The pressure of the saturation point P
in [bar].
And finally, the beta
value which is the mole fraction of the phase y
.
Pxy diagram for a mixture
We can use what we have learned to obtain a Pxy diagram for a mixture. Let’s try it out with the CO2 and n-butane mixture at 250 K.
[7]:
from yaeos import PengRobinson78
import matplotlib.pyplot as plt
import numpy as np
# Pure compounds properties
Tc = [304.2, 425.1] # critical temperature of CO2 and n-butane [K]
Pc = [73.8, 38.0] # critical pressure of CO2 and n-butane [bar]
w = [0.2236, 0.200164] # acentric factor of CO2 and n-butane [-]
model = PengRobinson78(Tc, Pc, w)
# Mole compositions from 0 to 1 for CO2
z_co2 = np.linspace(0.00001, 0.99999, 100)
z_butane = 1.0 - z_co2
t = 250 # K
# storing bubble and dew points
bubble_point_z_co2 = z_co2
dew_point_z_co2 = []
pressures = []
for z in zip(z_co2, z_butane):
sat_p = model.saturation_pressure(z=z, temperature=t, kind="bubble")
dew_point_z_co2.append(sat_p["y"][0])
pressures.append(sat_p["P"])
# Make diagram
plt.plot(bubble_point_z_co2, pressures, label="Bubble pressures")
plt.plot(dew_point_z_co2, pressures, label="Dew pressures")
plt.legend()
plt.title("Pxy CO2 - n-butane at 250 K")
plt.xlabel("XY-CO2")
plt.ylabel("Pressure [bar]")
plt.xlim(0, 1)
plt.ylim(0, 18)
[7]:
(0.0, 18.0)
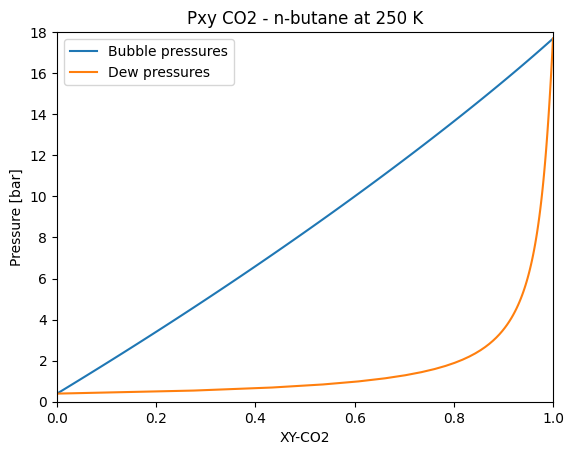
Flash calculation
Flash calculation are also supported by yaeos
. Let’s calculate a liquid-vapor flash at 250 K for the CO2 and n-butane mixture.
[8]:
# Pure compounds properties
n = [0.5, 0.5] # mole number of CO2 and n-butane [mol]
Tc = [304.2, 425.1] # critical temperature of CO2 and n-butane [K]
Pc = [73.8, 38.0] # critical pressure of CO2 and n-butane [bar]
w = [0.2236, 0.200164] # acentric factor of CO2 and n-butane [-]
model = PengRobinson78(Tc, Pc, w)
flash = model.flash_pt(z=[0.5, 0.5], pressure=4, temperature=250.0)
flash
[8]:
{'x': array([0.23809283, 0.76190717]),
'y': array([0.91415847, 0.08584153]),
'Vx': 0.0772038243107673,
'Vy': 4.9596098541608855,
'P': 4.0,
'T': 250.0,
'beta': 0.38739902408801924}
The result of the flash calculation is a Python dictionary with the same information as the saturation pressure calculation. We can plot the results in the Pxy diagram that we created before.
[9]:
plt.plot(bubble_point_z_co2, pressures, label="Bubble pressures")
plt.plot(dew_point_z_co2, pressures, label="Dew pressures")
# Flash result plot
x = flash["x"][0]
y = flash["y"][0]
p = flash["P"]
# guide to the eye
plt.plot([x, y], [p, p], linestyle="--", color="gray")
# flash compositions
plt.plot(
x,
p,
marker="s",
linestyle="none",
color="purple",
label="Flash: liquid phase",
)
plt.plot(
y,
p,
marker="s",
linestyle="none",
color="blue",
label="Flash: vapor phase",
)
# global composition
plt.plot(
0.5,
p,
marker="s",
linestyle="none",
color="red",
label="Global composition",
)
# Pxy diagram
plt.legend()
plt.title("Pxy CO2 - n-butane at 250 K")
plt.xlabel("XY-CO2")
plt.ylabel("Pressure [bar]")
plt.xlim(0, 1)
plt.ylim(0, 18)
[9]:
(0.0, 18.0)
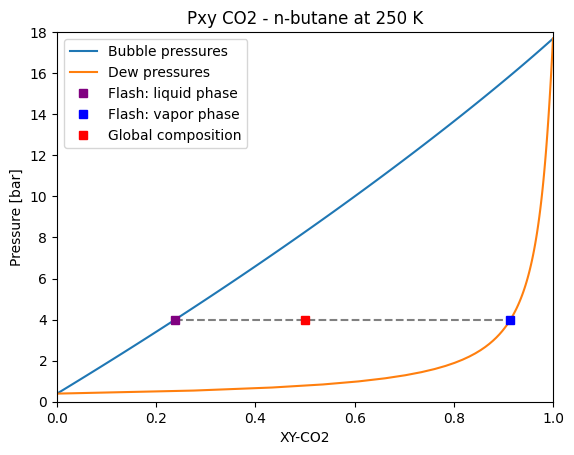
Phase envelopes
Most of times, calculating the whole lines of saturation points can be more efficient than calculating individual points while sweeping over a variable (for example, over a composition like we did before).
If the interest is to calculate the whole phase diagram of a mixture, then the phase envelope methods should be the go-to.
We first define a model that will be used for all the possible calculations.
[10]:
import yaeos
import matplotlib.pyplot as plt
# Pure compounds properties
n = [0.5, 0.5] # mole number of CO2 and n-butane [mol]
Tc = [304.2, 425.1] # critical temperature of CO2 and n-butane [K]
Pc = [73.8, 38.0] # critical pressure of CO2 and n-butane [bar]
w = [0.2236, 0.200164] # acentric factor of CO2 and n-butane [-]
model = yaeos.PengRobinson78(Tc, Pc, w)
PT phase envelope calculation
yaeos
also provides a method to calculate the 2 phase envelope of a mixture. Of course, we are going to keep using the CO2 and n-butane mixture, with a global composition [0.5, 0.5].
[11]:
env = model.phase_envelope_pt(
z=[0.5, 0.5], kind="bubble", max_points=600, t0=200.0, p0=1.0
)
Well, to make an envelope we have to specify a global composition z
. The kind
of the saturation point to start the envelope calculation (use “bubble” or “dew”). The max_point
parameters could not be set (default 300), is the maximum number of (P, T) points to calculate in the envelope. t0
is the initial guess for the temperature of the first saturation point of the envelope of kind: kind
(“bubble” in this case). And finally, p0
is the initial guess for the pressure of the
first saturation point of the envelope of kind: kind
.
The result of the envelope calculation is a Python dictionary with the keys:
[12]:
env.keys()
[12]:
dict_keys(['T', 'P', 'Tc', 'Pc', 'x', 'y', 'kinds'])
Being:
Ts: temperature points of the phase envelope
Ps: pressure points of the phase envelope
Tcs: critical temperatures of the phase envelope
Pcs: critical pressures of the phase envelope
We can plot the phase envelope as follow:
[13]:
# envelope
plt.plot(env["T"], env["P"], label="Envelope")
# Critical point
plt.plot(
env["Tc"],
env["Pc"],
marker="x",
linestyle="none",
color="red",
label="Critical point",
)
plt.legend()
plt.title("PT envelope CO2 (0.5) - n-butane (0.5)")
plt.xlabel("Temperature [K]")
plt.ylabel("Pressure [bar]")
[13]:
Text(0, 0.5, 'Pressure [bar]')
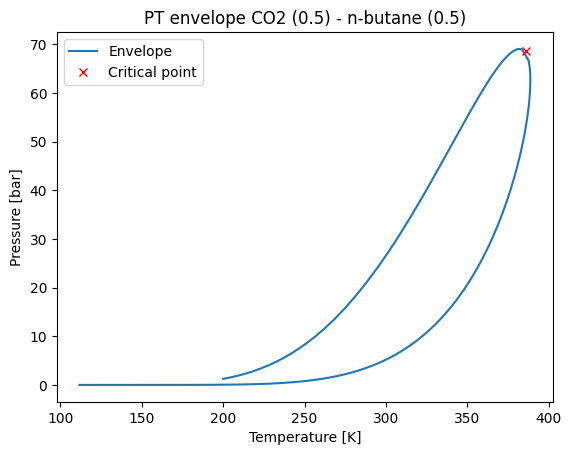
Px phase envelopes
Px phase envelopes can be calculated with the .phase_envelope_px
method
[14]:
z0 = [0, 1]
zi = [1, 0]
px = model.phase_envelope_px(z0=z0, zi=zi, temperature=380, p0=0.1, ds0=0.01)
plt.plot(px["x"][:, 0], px["P"], marker="o", label="Bubble")
plt.plot(px["y"][:, 0], px["P"], marker="o", label="Dew")
plt.xlabel(r"$x_1,y_1$")
plt.ylabel(r"Pressure [bar]")
plt.legend()
plt.xlim(0, 1)
plt.show()
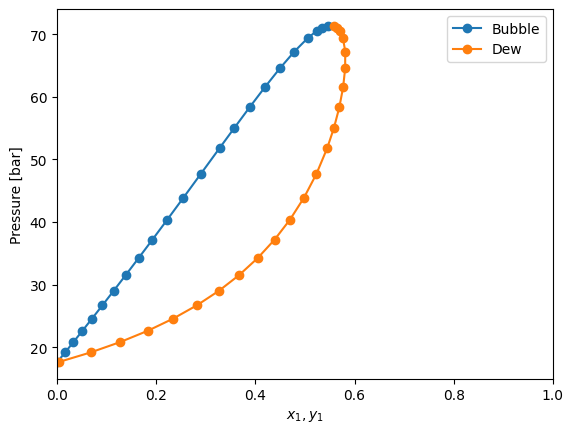
Tx phase envelopes
[15]:
# We start with pure n-butane
z0 = [0, 1]
# We reach to pure CO2
zi = [1, 0]
tx = model.phase_envelope_tx(z0=z0, zi=zi, pressure=1, t0=250, ds0=0.01, a0=0.01)
plt.plot(tx["x"][:, 0], tx["T"], marker="o", label="Bubble")
plt.plot(tx["y"][:, 0], tx["T"], marker="o", label="Dew")
plt.xlabel(r"$x_1,y_1$")
plt.ylabel(r"Temperature [K]")
plt.legend()
plt.xlim(0, 1)
plt.show()
WARN: possible bad root solving: -3902899490421.4790 1.0000000000000000
WARN: possible bad root solving: -23921311635284.699 1.0000000000000000
WARN: possible bad root solving: 7553330119836.1855 1.0000000000000000
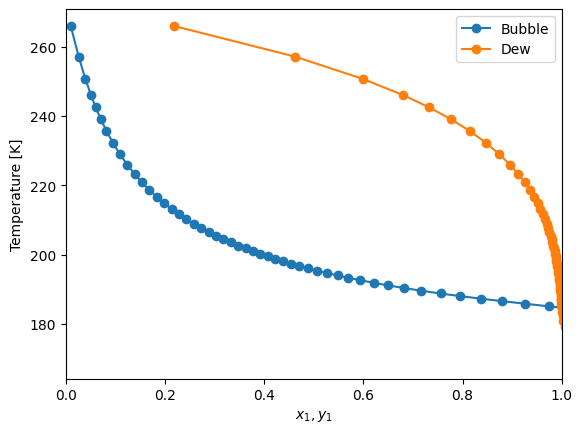
Ussually, it is better to start \(Tx\) envelopes from the light component instead of the heavy one. For this, we can change the initial value of a
and ds0
, to trace the line from right to left.
[16]:
z0 = [0, 1]
zi = [1, 0]
tx = model.phase_envelope_tx(
z0=z0, zi=zi, pressure=1, t0=200, a0=0.9999, ds0=-0.01
)
plt.plot(tx["x"][:, 0], tx["T"], marker="o", label="Dew")
plt.plot(tx["y"][:, 0], tx["T"], marker="o", label="Bubble")
plt.legend()
plt.xlim(0, 1)
plt.xlabel(r"$x_1,y_1$")
plt.ylabel(r"Temperature [K]")
plt.show()
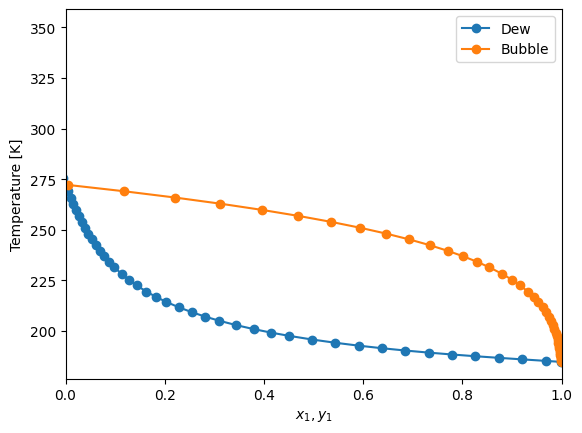
Critical lines
Calculation of critical points
[17]:
# Calculation of the critical point at z = [0.5, 0.5]
model.critical_point(z0=[0.5, 0.5])
[17]:
{'x': [array([0.5, 0.5])],
'Tc': 385.93041053482506,
'Pc': 68.20061554749196,
'Vc': 0.1848177989735669}
Calculation of critical lines
[18]:
cl = model.critical_line(z0=[1, 0], zi=[0, 1], a0=1e-2, s=1e-2)
plt.plot(cl["a"], cl["P"])
plt.xlabel(r"$x_2$")
plt.ylabel(r"$P [bar]$")
plt.show()
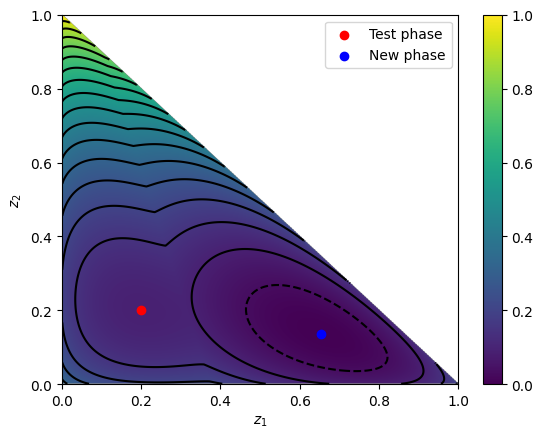
[19]:
plt.plot(cl["T"], cl["P"])
plt.xlabel(r"$T [K]$")
plt.ylabel(r"$P [bar]$")
[19]:
Text(0, 0.5, '$P [bar]$')
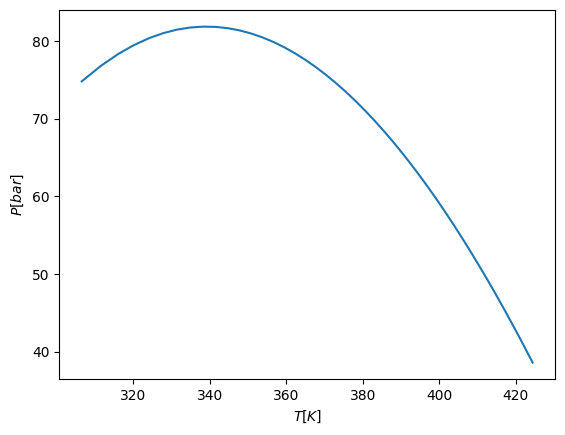
Stability analysis
In yaeos
it is possible to make stability analysis using the \(tm\) function defined by Michelsen and Møllerup
\begin{equation} tm = 1 + \sum_i w_i (\ln w_i + \ln \phi_i(\mathbf{w}) - \ln z_i - \ln \phi_i(\mathbf{z})) \end{equation}
where \(\mathbf{w}\) and \(\mathbf{z}\) are the mole numbers of the trial and test phase.
To show how to use the stability methods, we first define a system a find a region where there is phase equilibria. For this we obtain the critical constants with the chemicals
library and trace a phase envelope.
[20]:
import yaeos
import matplotlib.pyplot as plt
import numpy as np
import chemicals
components = ["methane", "propane"]
ids = [chemicals.CAS_from_any(name) for name in components]
Tc = [chemicals.critical.Tc(id) for id in ids]
Pc = [chemicals.critical.Pc(id) / 1e5 for id in ids]
w = [chemicals.acentric.omega(id) for id in ids]
z = [0.3, 0.7]
model = yaeos.PengRobinson78(Tc, Pc, w)
env = model.phase_envelope_pt(z, kind="bubble")
plt.plot(env["T"], env["P"], label="Envelope")
plt.scatter(env["Tc"], env["Pc"], color="red", label="Critical point")
plt.xlabel("T [K]")
plt.ylabel("P [bar]")
plt.show()
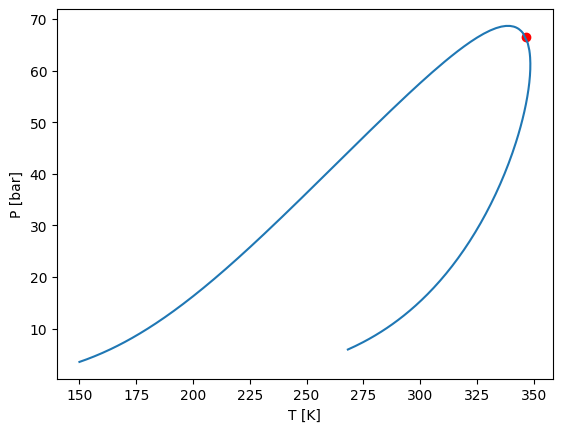
We can take a point of the phase envelope and calculate the \(tm\) function at pressures sligthly above and slightly below.
[21]:
# We take the high pressure point of the envelope closest to 250 K
T = 250
closest_index = np.where(abs(env["T"] - T) < 2)
Psat = max(env["P"][closest_index])
T_index = np.argmin(abs(env["P"] - Psat))
T = env["T"][T_index]
plt.plot(env["T"], env["P"], label="Envelope")
plt.scatter(T, Psat)
plt.scatter(T, Psat + 5)
plt.scatter(T, Psat - 5)
plt.ylabel("P [bar]")
plt.xlabel("T [K]")
plt.show()
Psat
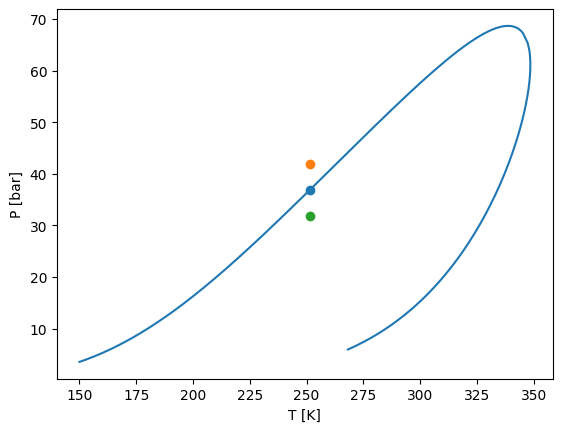
[21]:
36.862735486576916
How would the tm
function values compare in those three points? To know that, we will sweep on values of \(\mathbf{w_1}\) between \(0\) and \(1\) and plot the results.
[22]:
ws = np.linspace(0, 1, 100)
Ps = [Psat + 5, Psat, Psat - 5]
for P in Ps:
tms = []
for w1 in ws:
w = [w1, 1 - w1]
tm = model.stability_tm(z=z, w=w, pressure=P, temperature=T)
tms.append(tm)
plt.plot(ws, tms, label=f"{P:2f}")
plt.xlabel("w1")
plt.ylabel("tm")
plt.axhline(0, color="black", linestyle="--")
plt.legend(title="Pressure [bar]")
plt.title(
"""
Comparison of tm values at different pressures.
It is clear that above the saturation pressure the composition z is stable.
But, below the saturation pressure the composition z is unstable and another phase appears.
"""
)
plt.show()
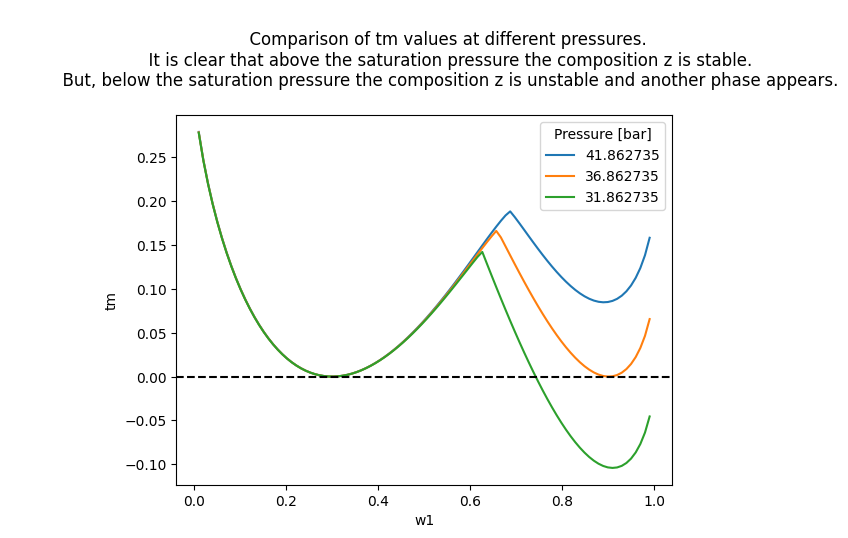
What if we have a ternary mixture?
[23]:
import yaeos
import matplotlib.pyplot as plt
import numpy as np
import chemicals
components = ["methane", "propane", "n-butane"]
ids = [chemicals.CAS_from_any(name) for name in components]
Tc = [chemicals.critical.Tc(id) for id in ids]
Pc = [chemicals.critical.Pc(id) / 1e5 for id in ids]
w = [chemicals.acentric.omega(id) for id in ids]
z = [0.2, 0.2, 0.6]
model = yaeos.PengRobinson78(Tc, Pc, w)
env = model.phase_envelope_pt(z, kind="bubble", max_points=1000)
plt.plot(env["T"], env["P"], label="Envelope")
plt.scatter(env["Tc"], env["Pc"], label="Critical point", color="red")
plt.ylabel("P [bar]")
plt.xlabel("T [K]")
plt.show()
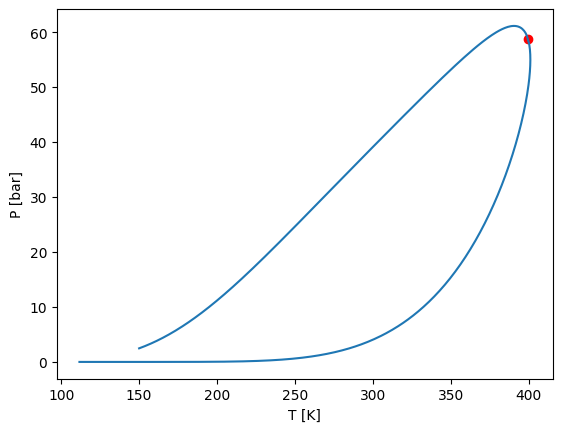
We can make the stability anaylisis sweeping on the composition of two components (using \(1 - w_1 - w_2\) for the remaining one) at a specified \(T\) and \(P\), and save the results.
[24]:
eps = 1e-15
npoints = 1000
w1s = np.linspace(0, 1, npoints)
w2s = np.linspace(0, 1, npoints)
tms = np.ones((npoints, npoints))
tms *= np.nan
P, T = 40, 350
minval = 0
for j, w1 in enumerate(w1s):
for i, w2 in enumerate(w2s):
w = [w1, w2, 1 - w1 - w2]
if w[-1] <= 0:
continue
tm = model.stability_tm(z=z, w=w, pressure=P, temperature=T)
if tm < minval:
w_min = w
minval = tm
tms[i, j] = tm
plt.imshow(tms, extent=(0, 1, 0, 1), origin="lower", aspect="auto")
plt.contour(
tms,
extent=(0, 1, 0, 1),
levels=[i / 10 for i in range(-25, 25)],
origin="lower",
colors="black",
)
plt.scatter(z[0], z[1], color="red", label="Test phase")
plt.scatter(w_min[0], w_min[1], color="blue", label="New phase")
plt.colorbar()
plt.legend()
plt.xlabel(r"$z_1$")
plt.ylabel(r"$z_2$")
plt.show()
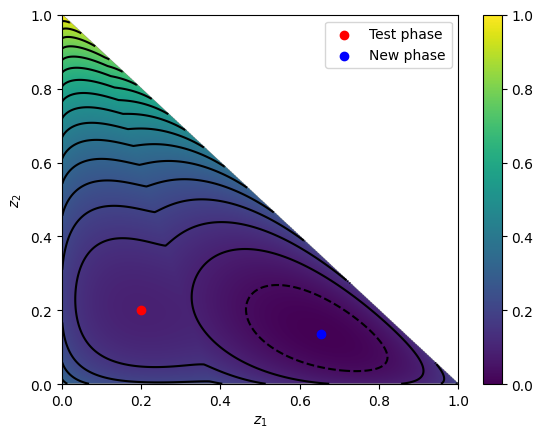
It is easy to notice that finding a minima by sweeping variables becomes harder and harder as more components are added. Luckily, in yaeos
there is also the method stability_analysis
that will use specific procedures to find the possible minima in an efficient way.
[25]:
minima, all_found_nimimas = model.stability_analysis(
z, pressure=P, temperature=T
)
minima
[25]:
{'w': array([0.65460664, 0.13648048, 0.20891288]), 'tm': -0.15793334532858538}
The stability_analysis
method returns a a tuple of dictionaries with two keys:
w
: The trial phase composition at the minimimum found value oftm
tm
: The miniumum value oftm
The first output of the tuple corresponds to the lower minima found, and the second one will a found minima for each initialization. Each initialization begins when starting from a pure component, so it will have the length of number of components.
[26]:
# Comparing the found w_min with the one obtained before by sweeping
# the composition space
print(minima["w"])
print(w_min)
[0.65460664 0.13648048 0.20891288]
[0.6546546546546547, 0.13613613613613612, 0.2092092092092092]
[27]:
minima
[27]:
{'w': array([0.65460664, 0.13648048, 0.20891288]), 'tm': -0.15793334532858538}
[28]:
all_found_nimimas
[28]:
{'tm': array([1.04563762, 1.17188022, 1.17188022]),
'w': array([[0.65460664, 0.13648048, 0.20891288],
[0.2 , 0.2 , 0.6 ],
[0.2 , 0.2 , 0.6 ]])}
Pure components saturation lines
[29]:
import yaeos
import matplotlib.pyplot as plt
import numpy as np
import chemicals
components = ["methane", "propane", "carbon dioxide", "limonene"]
ids = [chemicals.CAS_from_any(name) for name in components]
Tcs = [chemicals.critical.Tc(id) for id in ids]
Pcs = [chemicals.critical.Pc(id) / 1e5 for id in ids]
ws = [chemicals.acentric.omega(id) for id in ids]
model = yaeos.PengRobinson78(Tcs, Pcs, ws)
[30]:
for i, (Tc, Pc) in enumerate(zip(Tcs, Pcs)):
P, T, Vx, Vy = yaeos.yaeos_c.pure_saturation_line(model.id, i + 1, 1, 100)
plt.scatter(Tc, Pc)
plt.plot(T, P, label=components[i])
plt.xlim(0, max(Tcs) + 5)
plt.ylim(0, max(Pcs) + 5)
plt.xlabel("Temperature [K]")
plt.ylabel("Pressure [bar]")
plt.title("Pure components saturation lines")
plt.legend()
plt.show()
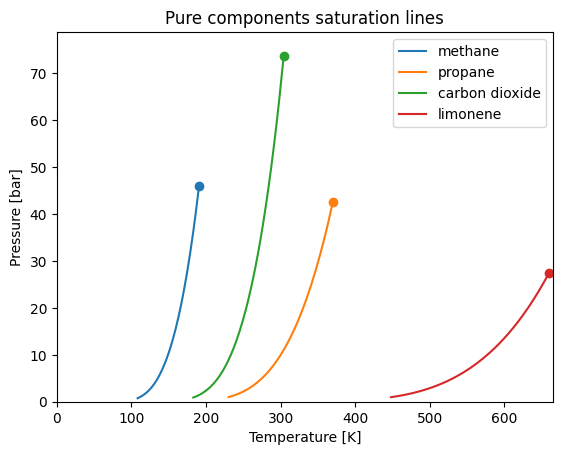
Three-phase envelopes
Assymetric systems can have three-phase behaviour. One of the common cases where three-phase behavoir can be expected is when we have a an open two-phase envelope and another two-phase envelope inside, this second envelope is actually an unstable line, but can be used to estimate the start of a three-phase line.
First let’s start by defining a model and calculating two two-phase envelopes. One starting from a dew point at low pressure and another starting from a bubble point at low temperature. Here it is possible to see the unstable bubble line.
[31]:
import yaeos
import numpy as np
import matplotlib.pyplot as plt
import chemicals
components = ["methane", "carbon dioxide", "n-eicosane"]
ids = [chemicals.CAS_from_any(name) for name in components]
Tc = [chemicals.critical.Tc(id) for id in ids]
Pc = [chemicals.critical.Pc(id) / 1e5 for id in ids]
w = [chemicals.acentric.omega(id) for id in ids]
kij = np.zeros((len(ids), len(ids)), order="F")
kij[0, 1] = kij[1, 0] = 0.1
kij[1, 2] = kij[2, 1] = 0.2
mixrule = yaeos.QMR(kij=kij, lij=0*kij)
model = yaeos.PengRobinson78(Tc, Pc, w, mixrule=mixrule)
z = [0.2, 0.4, 0.4]
dew = model.phase_envelope_pt(z, kind="dew", t0=300, p0=0.1, max_points=600)
bub = model.phase_envelope_pt(z, kind="bubble", t0=200, p0=10, max_points=100)
plt.plot(dew["T"], dew["P"])
plt.scatter(dew["Tc"], dew["Pc"])
plt.plot(bub["T"], bub["P"])
x, y = bub["T"][0], bub["P"][0]
plt.annotate("Unstable", (x+5, y+5), xytext=(x - 10, y + 50), arrowprops=dict(arrowstyle="->"))
plt.xlabel("Temperature [K]")
plt.ylabel("Pressure [bar]")
plt.show()
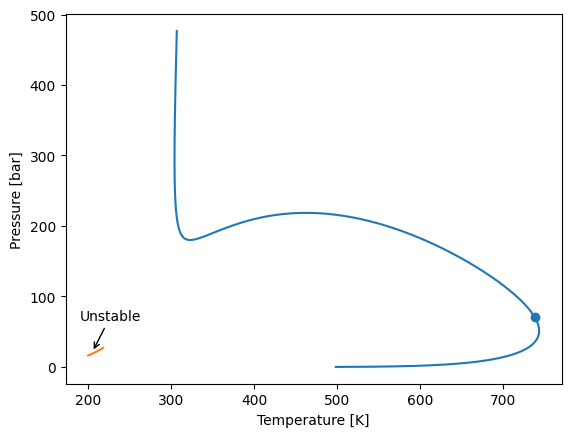
This unstable phase envelope can be used to start a three-phase envelope. First let’s see how a three-phase point is described.
A three-phase point can be described as the point where three-phases are in equilibrium. We can define this phases with the letters \(\mathbf{x}\), \(\mathbf{y}\) and \(\mathbf{w}\). In our case we are defining a saturation line, a line where one of the phases is incipient (it exists with a molar fraction of zero).
[32]:
T, P, y = bub["T"][0], bub["P"][0], bub["y"][0]
flash = model.flash_pt(z, temperature=T+50, pressure=P)
print(flash)
w = y
x = z
y = flash["y"]
beta = flash["beta"]
env3 = model.phase_envelope_pt3(z=z, x0=x, y0=y, w0=w, beta0=beta, t0=T, p0=P)
plt.plot(env3["T"], env3["P"], linestyle="--")
plt.title("Three-phase envelope")
plt.xlabel("Temperature [K]")
plt.ylabel("Pressure [bar]")
plt.show()
{'x': array([0.05824741, 0.17634038, 0.7654122 ]), 'y': array([3.55170062e-01, 6.44829938e-01, 1.59620402e-11]), 'Vx': 0.3814714130685887, 'Vy': 1.1204163454867, 'P': 16.376521867058983, 'T': 249.99999999999991, 'beta': 0.47740577194646566}
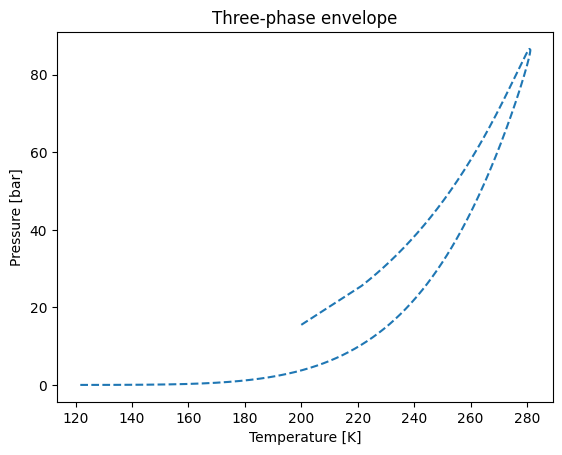
We now can plot the phase diagram including the three-phase envelope, and removing the unstable line.
[33]:
plt.plot(dew["T"], dew["P"], label="two-phase line")
plt.plot(env3["T"], env3["P"], linestyle="--", label="three-phase line")
plt.title("Full phase diagram")
plt.xlabel("Temperature [K]")
plt.ylabel("Pressure [bar]")
plt.legend()
plt.show()
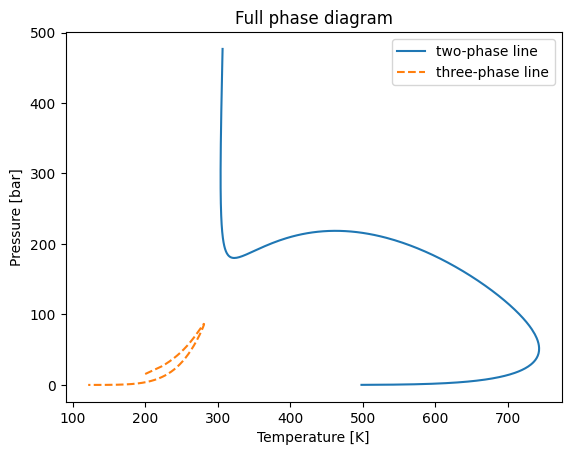